Fixing misconfigured Auto-Balanced Collector assignments
I’ve seen this issue pop up a lot in support so I figured this post may help some folks out. I just came across a ticket the other day so it’s fresh on my mind!
In order for Auto-Balanced Collector Groups (ABCG) to work properly, i.e. balance and failover, you have to make sure that the Collector Group is set to the ABCG and (and this is the important part) the Preferred Collector is set to “Auto Balance”. If it is set to an actual Collector ID, then it won’t get the benefits of the ABCG.
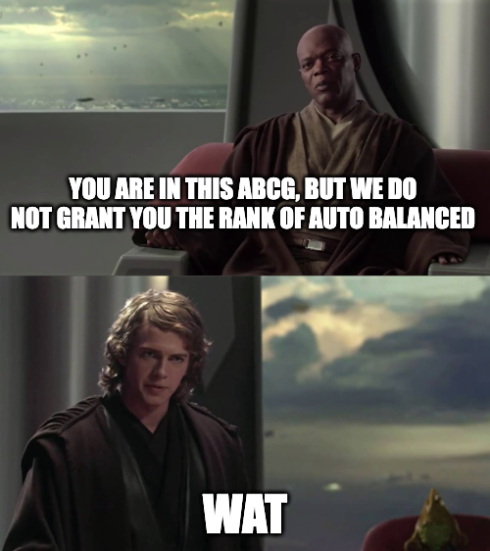
You want this, not that:
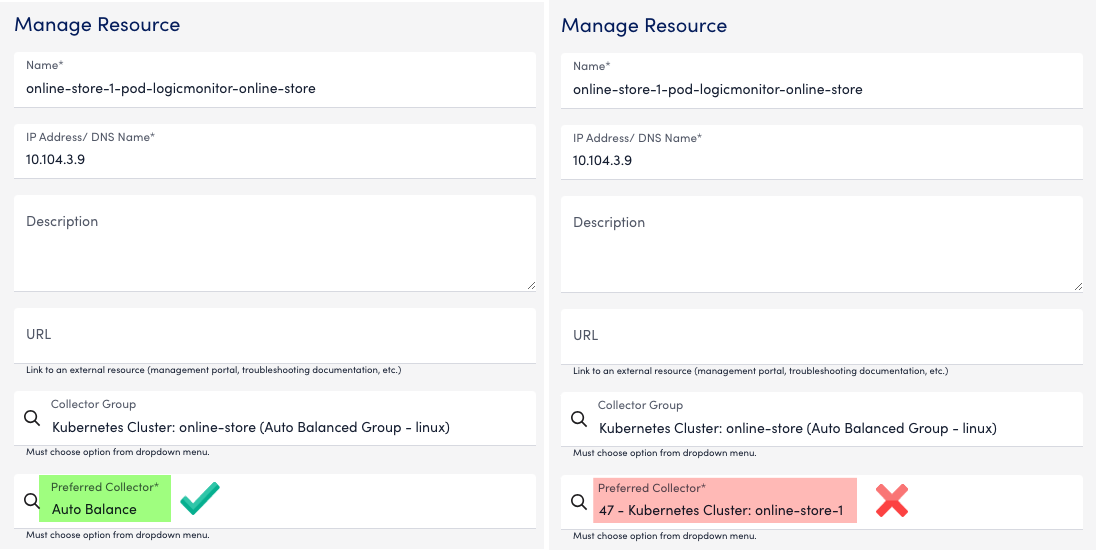
Ok, so that’s cool but now the real question is how do you fix this?
There’s not really a good way to surface in the portal all devices where this is misconfigured. It’s not a system property so a report or AppliesTo query won’t help here…
Fortunately, not all hope is lost! You can use the ✨API✨
When you GET a Resource/device, you will get back some JSON and what you want is for the autoBalancedCollectorGroupId
field to equal the preferredCollectorGroupId
field. If “Preferred Collector” is not “Auto Balance” and set to a ID, then autoBalancedCollectorGroupId
will be 0
.
Breaking it down step by step:
- First, get a list of all ABCG IDs
- https://www.logicmonitor.com/swagger-ui-master/api-v3/dist/#/Collector%20Groups/getCollectorGroupList
/setting/collector/groups?filter=autoBalance:true
- Then, with any given ABCG ID, you can filter a device list for all devices where there’s this mismatch
- https://www.logicmonitor.com/swagger-ui-master/api-v3/dist/#/Devices/getDeviceList
/device/devices?filter=autoBalancedCollectorGroupId:0,preferredCollectorGroupId:11
(where 11 is the ID of a ABCG)
- And now for each device returned, make a PATCH so that
autoBalancedCollectorGroupId
is now set topreferredCollectorGroupId
Here’s a link to the full script, written in Python for you to check out. I’ll also add it below in a comment since this is already getting long.
Do you have a better, easier, or more efficient way of doing this? I’d love to hear about it!